Step-by-Step Guide to .NET Transactional Data Handling
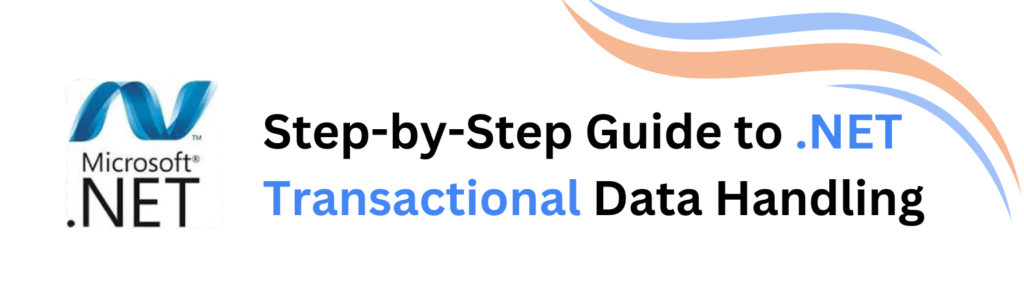
NET Transactional Data Handling: Ensuring Reliable Data Operations In modern software development, especially in enterprise-level applications, .NET transactional data handling plays a vital role in maintaining data consistency and integrity. When multiple operations interact with a database, there’s always a risk of partial updates or failures. Transactional handling ensures that a group of operations is executed as a single unit—commonly known as an atomic transaction. If one step fails, the entire transaction rolls back, preventing data corruption. Using tools like Entity Framework, developers can easily implement .NET transactional data handling with built-in support for transactions. This is particularly useful in scenarios like banking, order processing, and inventory management, where a failed operation must not leave the database in an inconsistent state. For example, transferring funds between accounts must either debit one account and credit the other—or do nothing at all. One of the core principles of transactional systems is ACID compliance—Atomicity, Consistency, Isolation, and Durability. The .NET framework, combined with SQL Server or any compatible database, supports these properties natively. Developers can create transaction scopes using classes like TransactionScope or through DbContext in Entity Framework, which makes it easy to commit or roll back changes based on the operation’s success. When handling multiple updates across tables or services, transactional data handling becomes even more critical. It not only simplifies error management but also improves system reliability. For example, in an e-commerce system, placing an order might involve updating inventory, logging the transaction, and notifying the user. If one step fails and transactions are not managed, you might end up with mismatched stock data or incomplete records. By integrating .NET transactional data handling into your application logic, you ensure your operations are safe, reversible, and reliable. This contributes to a smoother user experience, higher trust, and fewer data anomalies in production systems. In conclusion, whether you’re developing a simple CRUD application or a complex enterprise platform, mastering transactional handling in .NET is essential. With support from Entity Framework, you can implement robust, ACID-compliant logic that keeps your database operations safe and consistent.
The Complete Guide to .NET Core Development in 2025
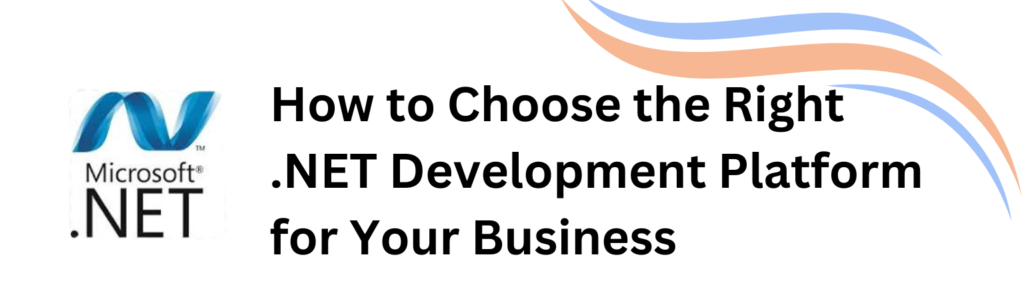
In today’s evolving tech landscape, developers seek frameworks that are fast, scalable, and cross-platform. One standout in this space is Microsoft’s modern .NET Core development platform, which continues to gain traction worldwide. Whether you’re a beginner looking to build web apps or a seasoned professional developing enterprise-grade software, understanding the power and flexibility of .NET Core is crucial in 2025 and beyond. What Is .NET Core? This open-source, cross-platform development framework maintained by Microsoft allows building applications that run on Windows, Linux, and macOS. Unlike its predecessor, which is Windows-only, it supports modern cloud-based and containerized applications. Its modularity, performance improvements, and compatibility with languages like C#, F#, and VB.NET make it a preferred choice for modern software development. Key Features of .NET Core Here are some standout features making this platform a favorite among developers: 1. Cross-Platform Compatibility Write your code once and run it anywhere—Windows, macOS, or Linux. Perfect for cloud-native apps and microservices. 2. High Performance Optimized runtime and Just-In-Time (JIT) compilation deliver fast execution, ideal for demanding applications. 3. Open Source and Community-Driven Developers can view, modify, and contribute to the source code. With Microsoft and community backing, the framework constantly evolves. 4. Modern Development Tools Integrated with Visual Studio, VS Code, and CLI tools for flexible, efficient workflows. 5. Microservices and Container Support Seamless compatibility with Docker and Kubernetes for cloud-native deployments. Why This Framework Matters in 2025 The software development ecosystem moves quickly, yet this platform remains relevant due to its versatility: Enterprise-level support for mission-critical apps Continuous innovation with regular updates Cloud readiness optimized for Azure, AWS, and Google Cloud Security-first design with frequent patches and built-in features Popular Use Cases This framework fits a wide variety of projects: Web APIs and backend services Real-time applications with SignalR Desktop apps with Windows Forms and WPF Cross-platform mobile apps via Xamarin and .NET MAUI Serverless applications with Azure Functions Machine learning integration with ML.NET How It Compares to the Classic .NET Framework Feature Modern Platform Classic Framework Platform Cross-platform Windows-only Open Source Yes Partially Performance High Moderate Cloud-ready Optimized Limited support Future Development Actively maintained Minimal updates For new projects, this platform is the recommended path. Getting Started: A Quick Guide Step 1: Install the SDK Download the latest software development kit from Microsoft’s official site. Step 2: Choose Your IDE Visual Studio, Visual Studio Code, or JetBrains Rider provide excellent support. Step 3: Create a New Project Run the following commands:Now you have a working web application. Step 4: Learn Core Concepts Focus on controllers, routing, dependency injection, middleware, and data access using Entity Framework Core. Tips for Better Development Adopt clean architecture principles for maintainability. Use dependency injection to improve testability. Implement robust logging and monitoring. Write unit and integration tests. Stay current with updates to enhance security and performance. Looking Ahead: Future Trends Upcoming enhancements promise even more power and flexibility: Continued unification of the .NET ecosystem with future versions Growing popularity of Blazor for client-side web apps using C# Expansion of .NET MAUI for truly cross-platform mobile and desktop apps Increased AI and machine learning integration Conclusion This modern Microsoft framework remains an excellent choice for building fast, scalable, and cross-platform applications in 2025. Its open-source nature, cross-platform flexibility, and enterprise-grade features make it ideal for everything from microservices to complex web apps.