How to Build a Scalable MERN Stack Application
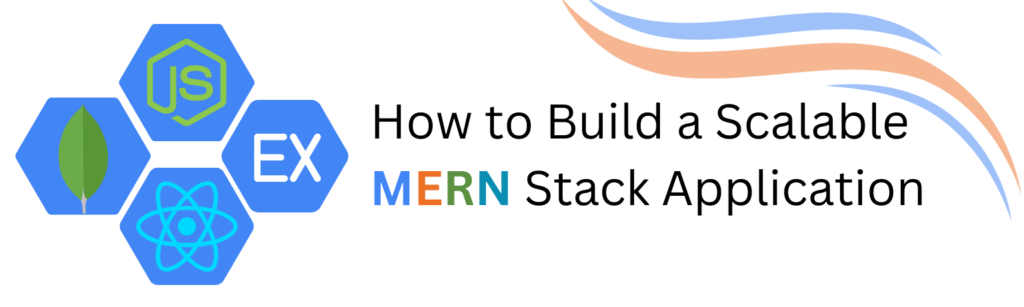
Introduction
As web applications evolve, scalability becomes a critical factor in ensuring that applications can handle increasing traffic and data loads efficiently. The MERN stack—comprising MongoDB,… Read More
What are React Design Patterns?
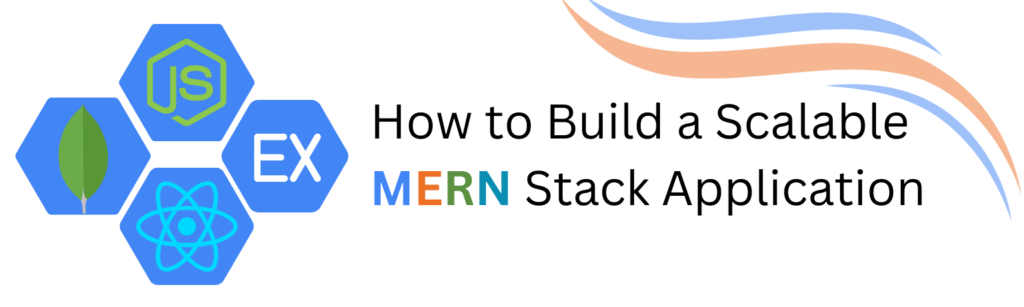
Have you considered the potential costs associated with having poorly organized code in your React application?
According to research conducted by Stack Overflow, the annual cost associated with managi… Read More